Php Function Cara Membuat Fungsi (function) Dengan P
Multiple Popup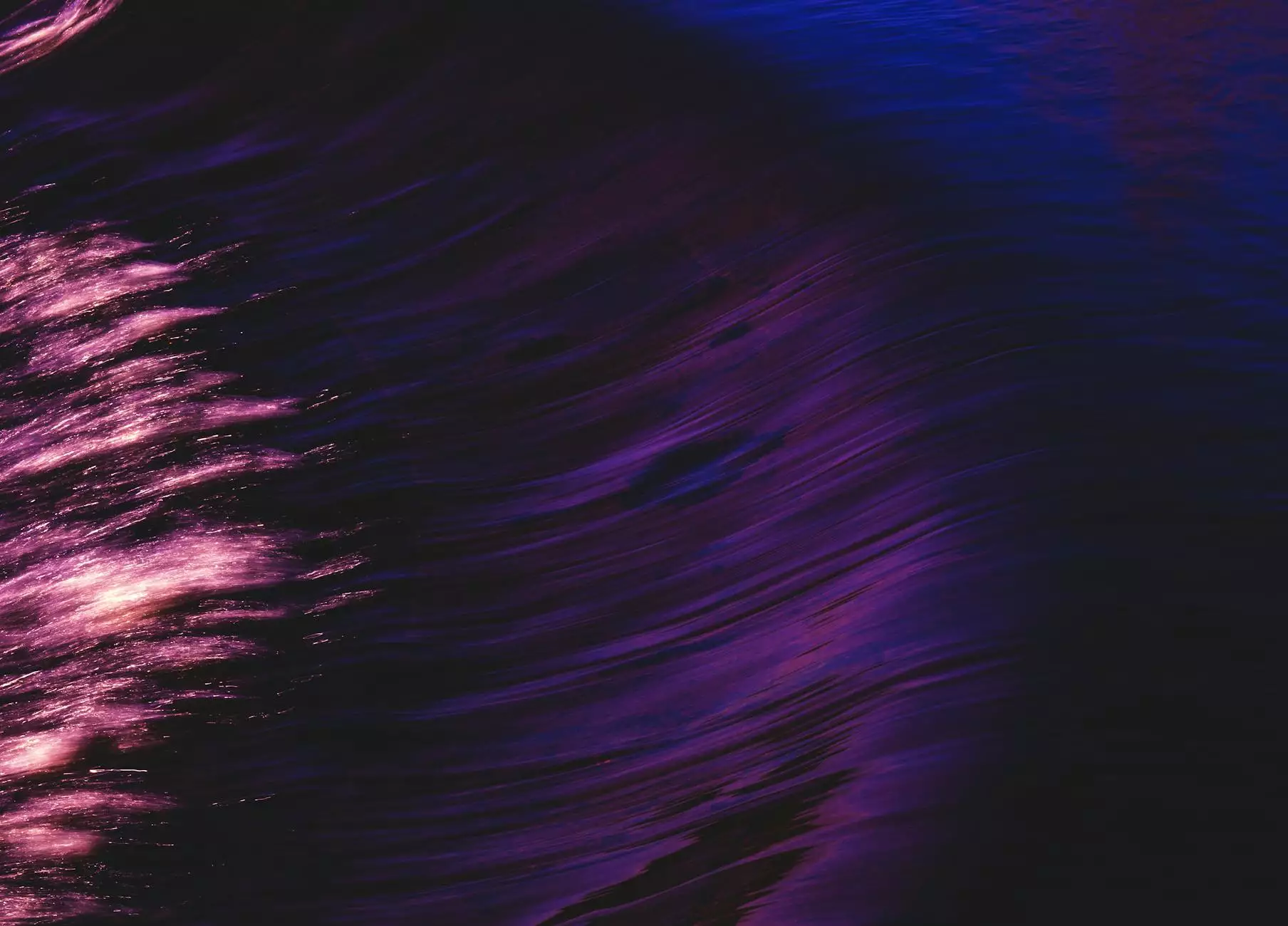
Introduction to PHP Functions
Welcome to our comprehensive guide on PHP functions! In this article, we will provide you with in-depth knowledge on how to create and utilize functions in PHP programming. Functions play a crucial role in code organization, reusability, and efficiency. By using functions effectively, you can enhance your PHP code and improve its overall quality.
Understanding Functions in PHP
Functions in PHP are blocks of code that can be defined and executed repeatedly throughout your program. They allow you to encapsulate a specific set of instructions and reuse them whenever needed. By using functions, you can break down complex tasks into smaller, more manageable parts.
A PHP function consists of a name, a set of parameters (optional), and a block of code. When the function is called, it executes the code within its body and can return a value if necessary. It's important to define functions with proper naming conventions that accurately describe their purpose and functionality.
Advantages of Using PHP Functions
There are several advantages to using functions in PHP programming:
- Code Reusability: Functions allow you to reuse blocks of code across multiple parts of your program, reducing redundancy and ensuring consistency.
- Modularity: By encapsulating code within functions, you can create modular code that is easier to understand, test, and maintain.
- Readability: Functions enhance the readability of your code by providing descriptive names and separating logic into smaller, more focused sections.
- Efficiency: Well-designed functions can improve the performance of your PHP code by reducing the duplication of code and optimizing execution.
- Scoping: Functions create their own scope, preventing variable and naming conflicts with other parts of your program.
Creating Your First PHP Function
Now that you understand the importance of functions in PHP, let's dive into creating your first function. To define a function, you use the function keyword followed by the function name and a pair of parentheses. Inside the parentheses, you can define the function's parameters (if any).
Here's an example of a simple PHP function that calculates the square of a given number:
function square($number) { return $number * $number; }In the above code snippet, we create a function called square that takes a single parameter $number. The function returns the square of the provided number by multiplying it with itself.
Calling and Implementing Functions
Once you have defined a function, you can call it from other parts of your PHP code. To call a function, simply write its name followed by a pair of parentheses. If the function accepts parameters, you pass the values within the parentheses.
Here's an example of calling the square function we defined earlier:
$result = square(5); echo $result; // Output: 25In the above code, we call the square function with the argument 5. The returned value, which is 25, is stored in the variable $result and then printed to the screen using the echo statement.
Tips for Writing Effective PHP Functions
To create high-quality and efficient PHP functions, consider the following tips:
- Use descriptive and meaningful function names that accurately represent the intended functionality.
- Keep your functions short and focused. A function should ideally perform a single task.
- Avoid global variables within functions. Instead, use function parameters for data input and return statements for data output.
- Document your functions using comments to provide clear explanations, parameter descriptions, and return value details.
- Perform error handling within functions to ensure proper execution and graceful error reporting.
Conclusion
In this guide, we have explored the world of PHP functions and their importance in PHP programming. By mastering the art of creating and implementing functions, you can significantly improve the structure, reusability, and efficiency of your PHP code. Remember to follow the best practices we discussed to write effective functions that enhance the overall quality of your applications.
Start leveraging the power of PHP functions today and take your programming skills to new heights!