Js Toggleclass JavaScript Class Method
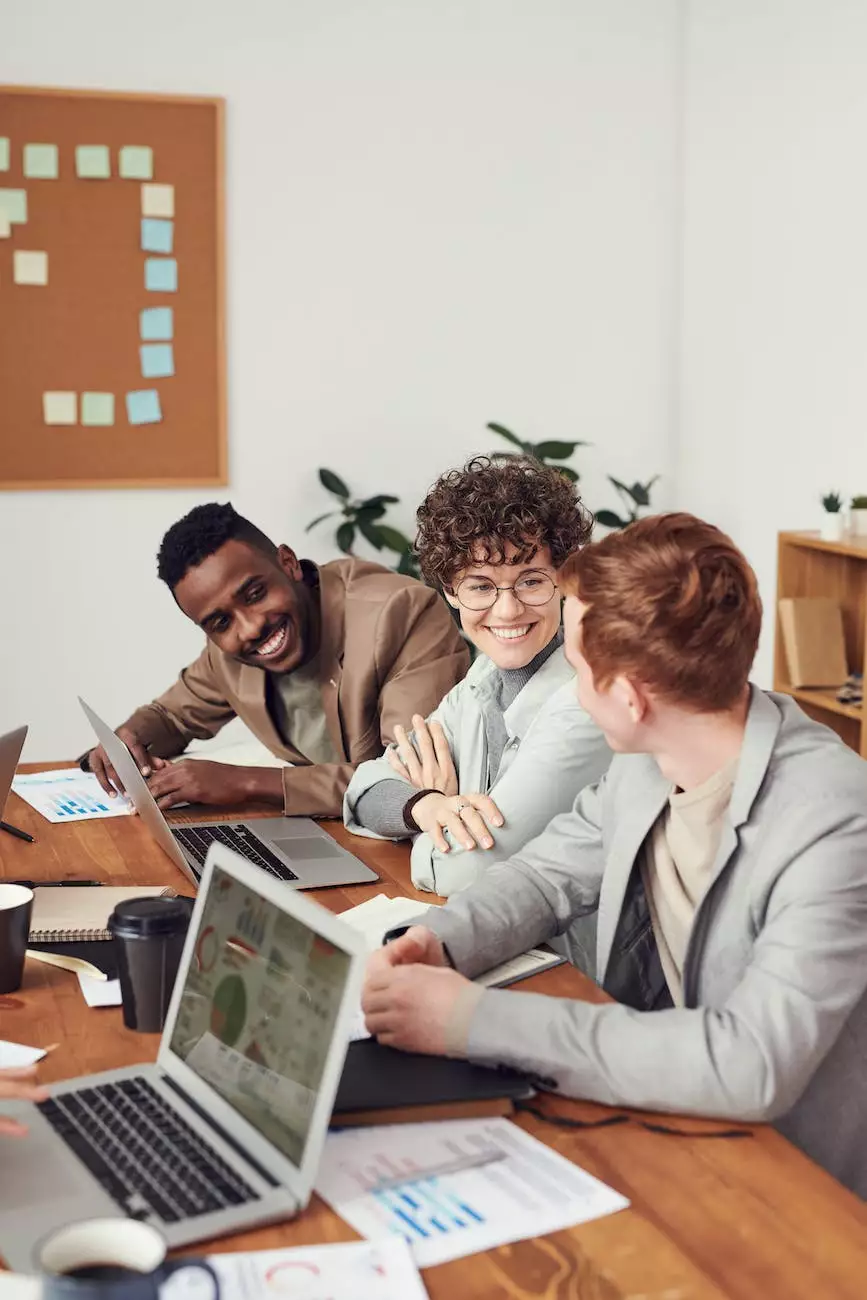
Introduction to Js Toggleclass JavaScript Class Method
The Js Toggleclass JavaScript Class Method is a powerful tool used for manipulating classes in JavaScript. This method allows you to easily add, remove, or toggle a class on an HTML element with just a few lines of code. Understanding how to use this method effectively can greatly enhance your web development capabilities.
Why You Should Use Js Toggleclass JavaScript Class Method
Using the Js Toggleclass JavaScript Class Method has several benefits:
- Simplified Class Manipulation: Instead of manually adding or removing classes using complex JavaScript logic, the Toggleclass method provides a simplified way to toggle classes on elements.
- Improved Code Readability: By using the method, your code becomes more readable and understandable, making it easier for other developers to collaborate and maintain your code.
- Enhanced User Interaction: Toggleclass enables you to create dynamic and interactive web interfaces by changing the appearance or behavior of elements based on user actions.
How to Use Js Toggleclass JavaScript Class Method
Implementing the Js Toggleclass JavaScript Class Method is straightforward. Here is an example:
const element = document.querySelector('.element'); element.classList.toggle('active');In the above example, we first select an element with the class name "element". We then use the Toggleclass method to toggle the class "active" on that element. This means that if the element already has the class "active", it will be removed, and if it doesn't have the class, it will be added.
Examples of Js Toggleclass JavaScript Class Method
Let's explore some practical examples to better understand how the Js Toggleclass JavaScript Class Method can be used in different scenarios:
Example 1: Toggling a Button's State
Assume you have a button element with the class "btn" and you want to toggle its active state when it is clicked. You can achieve this easily using the Toggleclass method:
const button = document.querySelector('.btn'); button.addEventListener('click', () => { button.classList.toggle('active'); });In the above example, whenever the button is clicked, it adds or removes the "active" class, effectively changing its appearance.
Example 2: Creating a Dark Mode Switch
Creating a dark mode switch on your website is a popular feature nowadays. Using Toggleclass, you can toggle between light and dark themes easily:
const toggleSwitch = document.querySelector('.toggle-switch'); const body = document.querySelector('body'); toggleSwitch.addEventListener('click', () => { body.classList.toggle('dark-mode'); });In the above example, we have a toggle switch with the class "toggle-switch". When the switch is clicked, it adds or removes the "dark-mode" class from the body element, effectively changing the theme of the entire website.
Conclusion
The Js Toggleclass JavaScript Class Method is a valuable tool for manipulating classes in your web applications. By leveraging this method, you can easily toggle classes, leading to enhanced interactivity and dynamic user experiences. Understanding how to utilize Js Toggleclass effectively opens up countless possibilities in your web development journey.
Stay tuned to Aicendo for more comprehensive tutorials, examples, and insights in the field of programming and developer software!